Logistic Regression
Logistic Regression is a classification algorithm that predicts the probability of a binary outcome based on one or more predictor variables. It uses the logistic function to map predictions to a probability between 0 and 1, making it suitable for binary classification tasks.
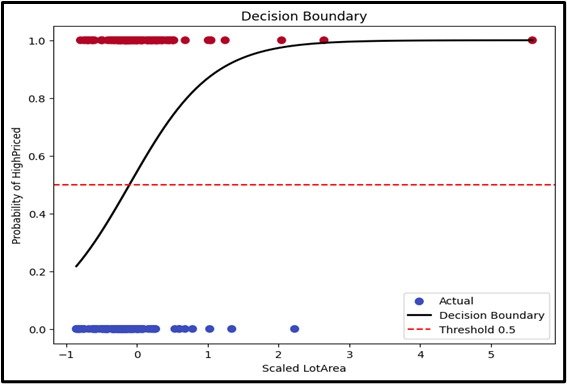
- Concept:Logistic Regression is a classification algorithm used to predict the probability of a binary outcome based on one or more predictor variables. It estimates the likelihood that an input belongs to a specific class by using the logistic (or sigmoid) function to map predictions to a
- Binary Classification:
It is designed for predicting binary outcomes, such as pass/fail or win/lose. - Probability Estimation:
The logistic function transforms the output into a probability value, which indicates the likelihood of the input belonging to a particular class.
- Applications: Logistic Regression is commonly used in:
- Medical Diagnosis: Predicting the presence or absence of a disease.
- Credit Scoring: Assessing the likelihood of a loan default.

Enhancing Model
Purpose:This algorithm predicts binary outcomes using the input feature.
Input Data: Numerical and categorical variables.
Output: Probability of the binary outcome (0 or 1).

Assumptions
Linear relationship between the logit of the outcome and the predictors, no multicollinearity, large sample size.

Use Case
You can use Logistic Regression when the dependent variable is binary. For example, predicting whether a passenger survived the Titanic disaster based on features like age, sex, class, and fare.
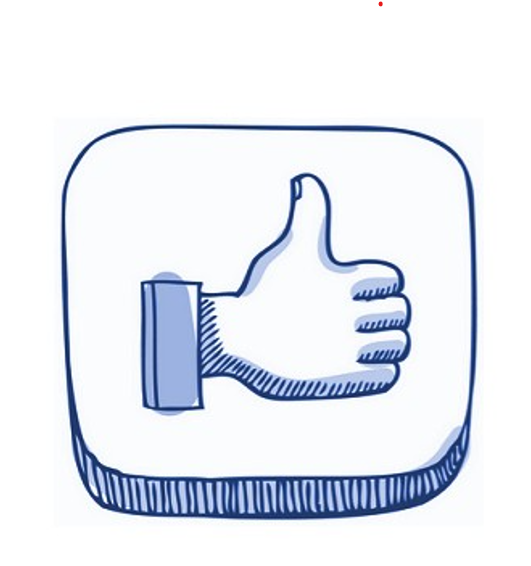
Advantages
- This is simple to implement and interpret.
- It provides probabilities and classifies observations.
- Computationally efficient and fast to train.
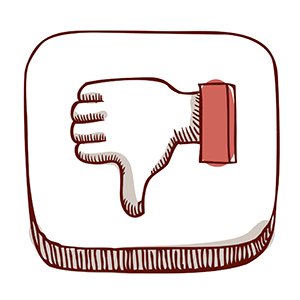
Disadvantages
- Assumes linearity between predictors and log odds.
- Sensitive to outliers and multicollinearity.
- May underperform with non-linear data.
Steps to Implement:
- Import necessary libraries like `numpy`, `pandas`, and `sklearn`.
- Load and preprocess data: Load the dataset, handle missing values, and prepare features and target variables.
- Split the data into training and testing sets using `train_test_split` to divide the data.
- From `sklearn.linear_model`, import and create an instance of `LogisticRegression`.
- Train the model: Use the `fit` method on the training data.
- Make predictions: Use the `predict` method on the test data.
- Evaluate the model: Check model performance using evaluation metrics like accuracy, precision, recall, F1 score, or the confusion matrix.
Ready to Explore?
Check Out My GitHub Code